今回の記事はDjangoのプロジェクト内でReactをDjangoでwebpack/babelを用いて実行する方法を見つけたのでそちらのまとめ記事になります。
では早速メイン記事に進みます。
Djangoプロジェクト作成
mkdir test_react
cd test_react
django-admin startproject test_django
cd test_django
python manage.py migrate #データの初期化
django-admin startapp frontend #アプリの作成
#テンプレート、staticの保管用フォルダ作成
mkdir -p ./frontend/src/components
mkdir -p ./frontend/{static,templates}/frontend
下記のようなtree構造になっています。
.
├── db.sqlite3
├── frontend
│ ├── __init__.py
│ ├── admin.py
│ ├── apps.py
│ ├── migrations
│ ├── models.py
│ ├── src
│ ├── static
│ ├── templates
│ ├── tests.py
│ └── views.py
├── manage.py
└── test_django
├── __init__.py
├── __pycache__
├── asgi.py
├── settings.py
├── urls.py
└── wsgi.py
ファイル内も編集していきます。
##############################
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'frontend', # 追加
]
from django.urls import path, include
urlpatterns = [
path('', include('frontend.urls')),
]
from django.urls import path
from . import views
urlpatterns = [
path('', views.index ),
]
from django.shortcuts import render
def index(request):
return render(request, 'frontend/index.html')
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bulma/0.6.2/css/bulma.min.css">
<title>Django DRF - React : Quickstart - Valentino G. - www.valentinog.com</title>
</head>
<body>
<section class="section">
<div class="container">
<div id="app" class="columns"><!-- React --></div>
</div>
</section>
</body>
{% load static %}
<script src="{% static "frontend/main.js" %}"></script>
</html>
最終的にhtmlの「main.js」が最終的にwebpackで「index.js」をコンパイルして「main.js」をstaticフォルダに出力します。その為、この記述はかなり重要ということです。
ではjavascriptのファイルを作成しておきます。
React・JavaScriptの記述
import React from "react";
import ReactDOM from "react-dom";
const App = () => {
return (
<div>
<p>React here!</p>
</div>
);
};
export default App;
ReactDOM.render(<App />, document.getElementById("app"));
「React here!」をhtmlのid=appに入れるというJavaScriptです。
index.jsをコンパイルして、main.jsとして出力するのでこちらのApp.jsをindex.jsで呼び出して使用します。
import App from "./components/App";
この記述がうまく反映されていないようなのですが、こちら問題ありませんのでそのまま進んでください。
Reactにwebpack・babelの設定
cd ..
test_reactのフォルダに移動
このフォルダでReactプロジェクトの必要なモジュールのインストール
npm init -y
package.jsonの作成
npm i webpack --save-dev
webpackのインストール
package-lock.json、node_modulesの作成
npm i webpack-cli --save-dev
webpackのnode_modulesフォルダの中のbinフォルダにwebpack-cliをインストール(気になる方はフォルダ開いて確認してください)
.
├── node_modules
├── package-lock.json
├── package.json
└── test_django
上記のようなtreeのはずです。
"scripts": {
"dev": "webpack --mode development ./test_django/frontend/src/index.js --output ./test_django/frontend/static/frontend/main.js",
"build": "webpack --mode production ./test_django/frontend/src/index.js --output ./test_django/frontend/static/frontend/main.js"
},
package.jsonのscriptsを上記のように記載します。
developmentの後ろがinput先でoutputが出力先になっており、index.jsをmain.jsにコンパイルする内容が記載されています。
devとbuildの違いは下記です。
npm run devは開発時に使います。出力のmain.jsがデバッグに備えて見やすいものになっています。
npm run buildは本番deploy時に使います。main.jsは圧縮された形で出力されます。
次にbabelのインストールです。
npm i @babel/core babel-loader @babel/preset-env @babel/preset-react babel-plugin-transform-class-properties --save-dev
node_modual内にbabelがインストールされています。
npm i react react-dom prop-types --save-dev
こちらも同様node_modual内にreact-domがインストールされています。
"devDependencies": {
"@babel/core": "^7.9.6",
"@babel/preset-env": "^7.9.6",
"@babel/preset-react": "^7.9.4",
"babel-loader": "^8.1.0",
"babel-plugin-transform-class-properties": "^6.24.1",
"prop-types": "^15.7.2",
"react": "^16.13.1",
"react-dom": "^16.13.1",
"webpack": "^4.43.0",
"webpack-cli": "^3.3.11"
}
上記のようにインストール時に–save-devを付けているので、package.jsonのdevDependenciesにインストールしたものが記述されています。(これはすでに記載されているので追加で記載する必要ばなし)
次にbabelの設定をしていきます。
現在のディレクトリで.babelrcファイルを作成し下記を記述。
{
"presets": [
"@babel/preset-env", "@babel/preset-react"
],
"plugins": [
"transform-class-properties"
]
}
次にwebpack.config.jsもこのディレクトリ上に作成し、下記を記述。
module.exports = {
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: "babel-loader"
}
}
]
}
};
これで完成したものをコンパイルしてみましょう。
npm run build
成功すればDjangoのstaticフォルダ内にmain.jsが出力されます。
Djangoでローカルサーバ起動
cd test_django
python manage.py runserver
こちらで確認してみてください。
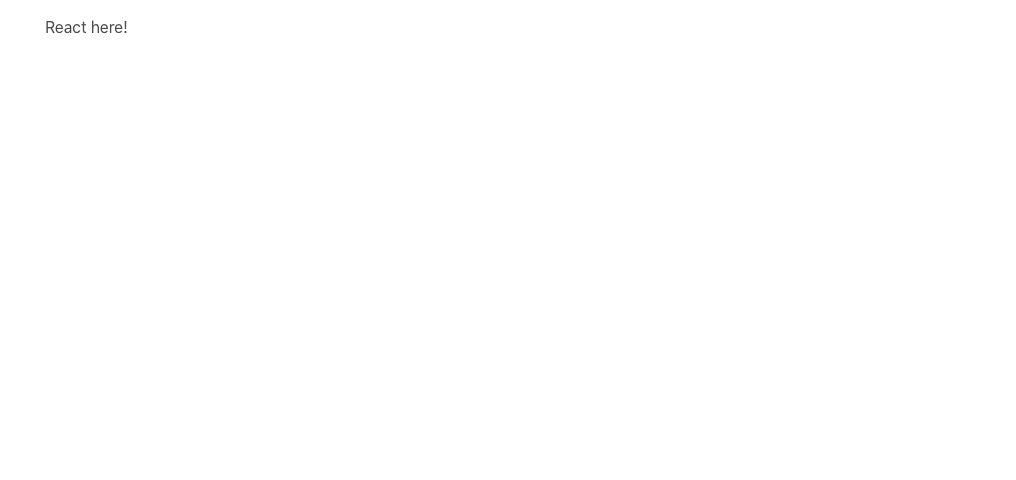
上記のような記述でOKです。
今回の記述は以上です。TypeScriptでの実装もできるような気もするので時間のある時にやって見ます。他にもDjangoやTypeScriptの記事を多数記載しているので気になる方は是非参考にしてみてください。
コメント
[…] 【Django】DjangoでReactを使いhtmlに表示させる方法。「webpack/babel使用」 カテゴリーTypeScript […]